Sanity with Next.js
Next.js is a popular framework for building dynamic and static websites, and it pairs well with Sanity’s structured content model. Here’s how businesses can leverage this integration to build scalable, performance-driven applications.
Install sanity client
Begin by installing the Sanity client to allow your Next.js application to fetch data from your Sanity project:
npm install @sanity/client
Configure the Sanity client
Create a sanityClient.js file in your project:
import sanityClient from '@sanity/client';
const client = sanityClient({
projectId: 'your-project-id', // Your Sanity project ID
dataset: 'your-dataset-name', // Your dataset name
apiVersion: '2021-05-21', // API version
useCdn: true, // Enable CDN for faster response times
});
export default client;
Fetch data in Next.js
Use the following example to fetch and display blog posts:
import client from '../sanityClient';
export async function getStaticProps() {
const query = `*[_type == "post"]{
title,
slug,
mainImage{asset->{url}},
body
}`;
const posts = await client.fetch(query);
return {
props: { posts },
revalidate: 60,
};
}
const Blog = ({ posts }) => (
<div>
{posts.map((post) => (
<article key={post.slug.current}>
<h2>{post.title}</h2>
<img src={post.mainImage.asset.url} alt={post.title} />
</article>
))}
</div>
);
export default Blog;
This example demonstrates how content stored in Sanity is dynamically fetched and rendered in a Next.js application.
How WordPress compares
While Sanity’s integration with frameworks like Next.js is powerful, WordPress provides an alternative that’s often more accessible:
Pre-built ecosystem
Over 13,000 free themes and over 60,000 plugins minimize the need for custom coding, enabling faster project launches.
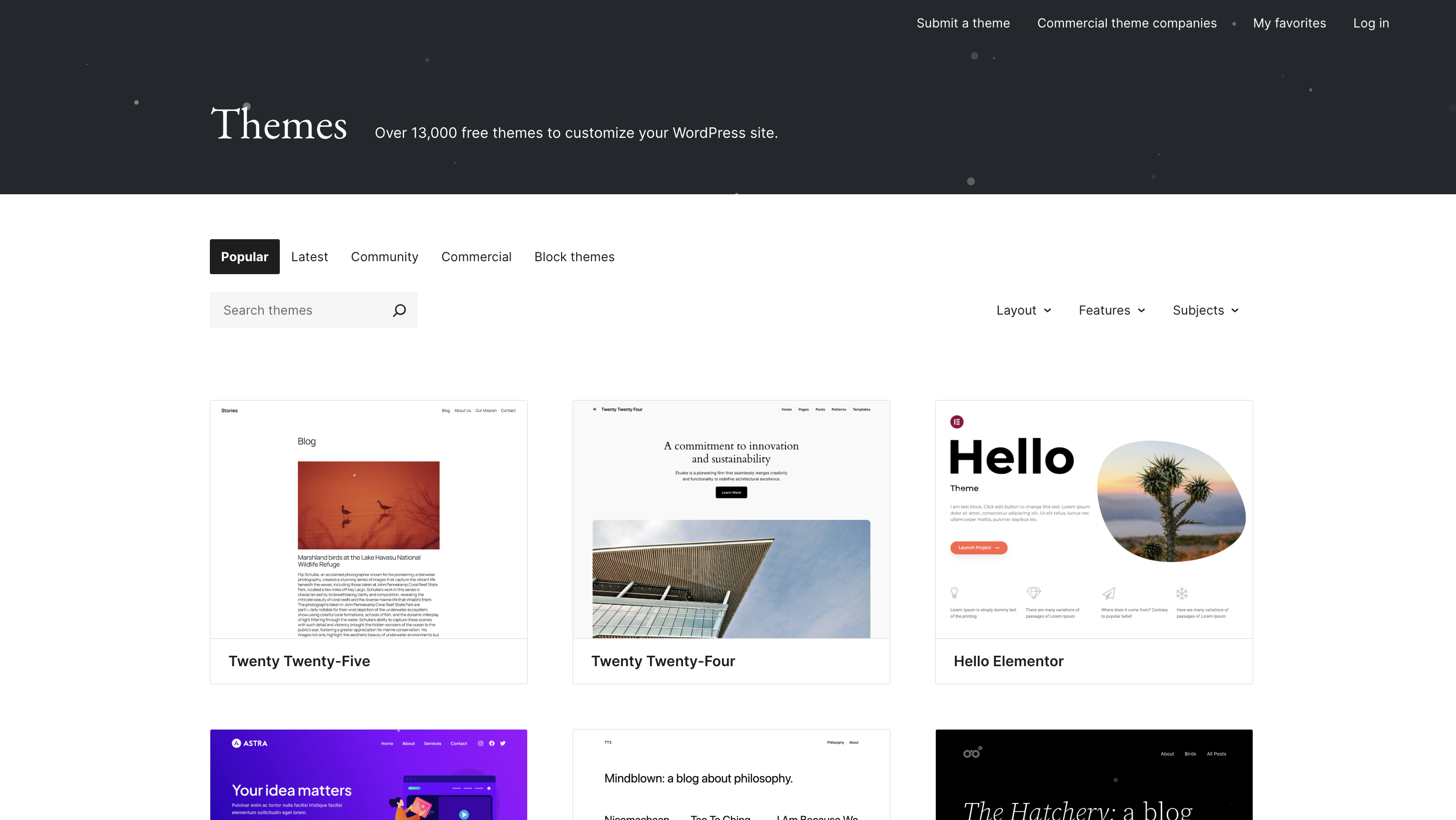
Robust API support ensures WordPress can work seamlessly with frameworks like Next.js, Gatsby, and beyond.
Hybrid approach
Start with WordPress’ traditional monolithic setup and transition to a headless architecture as your business grows, reducing disruption and development costs.
For businesses seeking a balance between flexibility and ease of use, WordPress’ framework-agnostic approach offers a compelling alternative. Read more about it here.